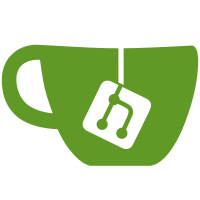
In some (most) cases, disabled chars may not be specified. if this is the case, they should be omitted.
97 lines
5.0 KiB
Go
97 lines
5.0 KiB
Go
package pwgenerator
|
|
|
|
import (
|
|
"encoding/xml"
|
|
"time"
|
|
)
|
|
|
|
// pwHash is an explicit hash algorithm.
|
|
type pwHash uint8
|
|
|
|
// cryptoShuffler is used to shuffle a slice in a cryptographically sane way.
|
|
type cryptoShuffler struct{}
|
|
|
|
// Char is implemented as a rune.
|
|
type Char rune
|
|
|
|
// CharSet is a collection of characters (Char) but with additional methods (e.g. sort.Sort interface conformance).
|
|
type CharSet []Char
|
|
|
|
// GenOpts controls what kind of (and how many) password(s) should be generated.
|
|
type GenOpts struct {
|
|
/*
|
|
Some references below contain a reference to the character's hex ASCII.
|
|
If you need the decimal/octal/etc. reference instead, you can cross-reference
|
|
it via https://square-r00t.net/ascii.html.
|
|
*/
|
|
/*
|
|
HumanOnly avoids visually-ambiguous characters if enabled, ensuring a more readable, visually-distinguishable,
|
|
and accessible but MUCH smaller password character space (from about 220 characters to ), thus much less secure.
|
|
|
|
TODO: Get feedback on this. I feel like I'm trimming out WAY too many chars.
|
|
*/
|
|
// HumanOnly bool `json:"do_human_readable" toml:"do_human_readable" yaml:"Human-Readable Only" xml:"doHumanReadable,attr"`
|
|
// Alpha is true if letters (0x41 to 0x5a, 0x61 to 0x7a) should be included.
|
|
Alpha bool `json:"do_alpha" toml:"do_alpha" yaml:"Letters" xml:"doAlpha,attr"`
|
|
// Numeric is true if numbers (0x30 to 0x39) should be included.
|
|
Numeric bool `json:"do_numeric" toml:"do_numeric" yaml:"Numbers" xml:"doNumeric,attr"`
|
|
// Symbols is true if non-alphanumeric characters (between 0x21 and 0x7e) should be included.
|
|
Symbols bool `json:"do_symbols" toml:"do_symbols" yaml:"Symbols" xml:"doSymbols,attr"`
|
|
// ExtendedSymbols is true if non-alphanumeric characters in the "extended ASCII" set (0x80 to 0xff) should be included.
|
|
ExtendedSymbols bool `json:"do_extended" toml:"do_extended" yaml:"Extended Symbols" xml:"doExtendedSymbols,attr"`
|
|
// CountUpper specifies how many uppercase letters (0x41 to 0x5a) should be specified at a minimum.
|
|
CountUpper uint `json:"uppers" toml:"uppers" yaml:"Number of Uppercase Letters" xml:"numUppers,attr"`
|
|
// CountLower specifies how many lowercase letters (0x61 to 0x7a) should be specified at a minimum.
|
|
CountLower uint `json:"lowers" toml:"lowers" yaml:"Number of Lowercase Letters" xml:"numLowers,attr"`
|
|
// CountNumbers specifies how many numbers (0x30 to 0x39) should be specified at a minimum.
|
|
CountNumbers uint `json:"numbers" toml:"numbers" yaml:"Number of Numeric Characters" xml:"numNumeric,attr"`
|
|
// CountSymbols specifies how many symbols (0x21 to 0x7e) should be specified at a minimum.
|
|
CountSymbols uint `json:"symbols" toml:"symbols" yaml:"Number of Symbols" xml:"numSymbols,attr"`
|
|
// CountExtended specifies how many extended symbols (0x80 to 0xff) should be specified at a minimum.
|
|
CountExtended uint `json:"extended" toml:"extended" yaml:"Number of Extended Symbols" xml:"numXSymbols,attr"`
|
|
// DisabledChars includes characters that should NOT be included from the above selection options.
|
|
DisabledChars CharSet `json:"disabled_chars,omitempty" toml:"disabled_chars,omitempty" yaml:"Disabled CharSet,omitempty" xml:"disabledChars,omitempty"`
|
|
// LengthMin specifies how long (in characters/bytes) each password should be at minimum. Use 0 for no minimum.
|
|
LengthMin uint `json:"length_min" toml:"length_min" yaml:"Minimum Length" xml:"minLen,attr"`
|
|
/*
|
|
LengthMax specifies the maximum length for each password. Set to 0 for no limit
|
|
(the language has a hard limit of 18446744073709551615; this is limited to 256 for performance reasons).
|
|
*/
|
|
LengthMax uint `json:"length_max" toml:"length_max" yaml:"Maximum Length" xml:"maxLen,attr"`
|
|
// Count specifies how many passwords to generate. If 0, the default is 1.
|
|
Count uint `json:"count" toml:"count" yaml:"Number of Passwords" xml:"genCount,attr"`
|
|
// explicitCharset is the collection of acceptable characters as explicitly defined by the caller, if any.
|
|
explicitCharset CharSet
|
|
}
|
|
|
|
// PwCollection contains the full series of generated passwords.
|
|
type PwCollection struct {
|
|
XMLName xml.Name `json:"-" yaml:"-"`
|
|
Passwords []*PwDef `json:"password_defs" yaml:"Password Definitions" xml:"passwordDefs"`
|
|
}
|
|
|
|
// PwDef contains a generated password and related metadata.
|
|
type PwDef struct {
|
|
XMLName xml.Name `json:"-" yaml:"-"`
|
|
Password string `json:"password" yaml:"Password" xml:"password,attr"`
|
|
Generated time.Time `json:"generated" yaml:"Generated" xml:"generated,attr"`
|
|
Hashes []PwHashDef `json:"hashes,omitempty" yaml:"Hashes,omitempty" xml:"hashes,omitempty"`
|
|
// Hashes []PwHashDef `json:"hashes" yaml:"Hashes" xml:"hashes"`
|
|
}
|
|
|
|
// PwHashDef defines a hash for a PwDef (once we implement it). Might want to move it to the pwhash subpackage.
|
|
type PwHashDef struct {
|
|
XMLName xml.Name `json:"-" yaml:"-"`
|
|
HashType string `json:"hash_algo" yaml:"Hash Algorithm" xml:"hashAlgo,attr"`
|
|
HashString string `json:"hash" yaml:"Hash" xml:",chardata"`
|
|
}
|
|
|
|
// selectFilter is used to include specified number of characters.
|
|
type selectFilter struct {
|
|
upperCounter uint
|
|
lowerCounter uint
|
|
numberCounter uint
|
|
symbolCounter uint
|
|
extendedCounter uint
|
|
}
|