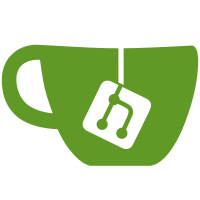
FIXES: * There was an edge case where a raw body for HTML would potentially result in a nil byte slice exception. This has been fixed. (I don't even know if it was possible, I just made sure it wasn't.) * The links browser is now explicitly returned as HTML and properly detected as a "browser". * Hyperlinks for links, w3m added to Usage page. * Content-Type is now always set correctly; there were cases where it was improperly returning e.g. text/plain for JSON.
94 lines
2.2 KiB
Go
94 lines
2.2 KiB
Go
package server
|
|
|
|
import (
|
|
`embed`
|
|
`encoding/json`
|
|
`encoding/xml`
|
|
`html/template`
|
|
`os`
|
|
`syscall`
|
|
|
|
sysdUtil `github.com/coreos/go-systemd/util`
|
|
`github.com/goccy/go-yaml`
|
|
)
|
|
|
|
const (
|
|
convertTag string = "uaField"
|
|
prettyTag string = "renderName"
|
|
baseTitle string = "r00t^2 Client Info Revealer"
|
|
titleSep string = " || "
|
|
xmlHdrElem string = "header"
|
|
xmlHdrElemName string = "name"
|
|
xmlHdrVal string = "value"
|
|
nilUaFieldStr string = "(N/A)"
|
|
trueUaFieldStr string = "Yes"
|
|
falseUaFieldStr string = "No"
|
|
dfltIndent string = " "
|
|
httpRealHdr string = "X-ClientInfo-RealIP"
|
|
)
|
|
|
|
var (
|
|
//go:embed "tpl"
|
|
tplDir embed.FS
|
|
tpl *template.Template = template.Must(
|
|
template.New("").
|
|
Funcs(
|
|
template.FuncMap{
|
|
"getTitle": getTitle,
|
|
},
|
|
).ParseFS(tplDir, "tpl/*.tpl"),
|
|
)
|
|
)
|
|
|
|
// Signal traps
|
|
var (
|
|
stopSigs []os.Signal = []os.Signal{
|
|
syscall.SIGQUIT,
|
|
os.Interrupt,
|
|
syscall.SIGTERM,
|
|
}
|
|
reloadSigs []os.Signal = []os.Signal{
|
|
syscall.SIGHUP,
|
|
// We also add stopSigs so we trigger the Reload loop to close. TODO.
|
|
syscall.SIGQUIT,
|
|
os.Interrupt,
|
|
syscall.SIGTERM,
|
|
}
|
|
isSystemd bool = sysdUtil.IsRunningSystemd()
|
|
)
|
|
|
|
// media/MIME types
|
|
const (
|
|
mediaJSON string = "application/json"
|
|
mediaXML string = "application/xml"
|
|
mediaYAML string = "application/yaml"
|
|
mediaHTML string = "text/html"
|
|
// TODO: plain/text? CSV? TOML?
|
|
)
|
|
|
|
var (
|
|
// mediaNoIndent covers everything (except HTML).
|
|
mediaNoIndent map[string]func(obj any) (b []byte, err error) = map[string]func(obj any) (b []byte, err error){
|
|
mediaJSON: json.Marshal,
|
|
mediaXML: xml.Marshal,
|
|
mediaYAML: yaml.Marshal,
|
|
// HTML is handled explicitly.
|
|
}
|
|
// mediaIndent only contains MIME types that support configured indents.
|
|
mediaIndent map[string]func(obj any, pfx string, indent string) (b []byte, err error) = map[string]func(obj any, pfx string, indent string) (b []byte, err error){
|
|
mediaJSON: json.MarshalIndent,
|
|
mediaXML: xml.MarshalIndent,
|
|
}
|
|
// valid MIMEs.
|
|
okAcceptMime []string = []string{
|
|
mediaJSON,
|
|
mediaXML,
|
|
mediaYAML,
|
|
mediaHTML,
|
|
}
|
|
// These are actually HTML.
|
|
htmlOverride map[string]bool = map[string]bool{
|
|
"Links": true,
|
|
}
|
|
)
|