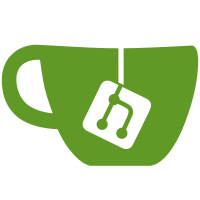
ADDED: * cryptpartse.TlsUri now has methods to returned dialed net.Conn and tls.Conn, or can use WithConn to add TLS to an already-dialed net.Conn.
135 lines
3.6 KiB
Go
135 lines
3.6 KiB
Go
package cryptparse
|
|
|
|
import (
|
|
`crypto/tls`
|
|
|
|
`github.com/go-playground/validator/v10`
|
|
)
|
|
|
|
var (
|
|
tlsVerNmToUint map[string]uint16
|
|
tlsCipherNmToUint map[string]uint16
|
|
tlsCurveNmToCurve map[string]tls.CurveID
|
|
)
|
|
|
|
const (
|
|
MaxTlsCipher uint16 = tls.TLS_ECDHE_ECDSA_WITH_CHACHA20_POLY1305_SHA256
|
|
MaxCurveId tls.CurveID = tls.X25519 // 29
|
|
MinTlsVer uint16 = tls.VersionSSL30
|
|
MaxTlsVer uint16 = tls.VersionTLS13
|
|
DefaultNetType string = "tcp"
|
|
)
|
|
|
|
// TlsUriParam* specifiy URL query parameters to parse a tls:// URI, and are used by TlsUri methods.
|
|
const (
|
|
/*
|
|
TlsUriParamCa specifies a path to a CA certificate PEM-encded DER file.
|
|
|
|
It may be specified multiple times in a TLS URI.
|
|
*/
|
|
TlsUriParamCa string = "pki_ca"
|
|
/*
|
|
TlsUriParamCert specifies a path to a client certificate PEM-encded DER file.
|
|
|
|
It may be specified multiple times in a TLS URI.
|
|
*/
|
|
TlsUriParamCert string = "pki_cert"
|
|
/*
|
|
TlsUriParamKey specifies a path to a private key as a PEM-encded file.
|
|
|
|
It may be PKCS#1, PKCS#8, or PEM-encoded ASN.1 DER EC key.
|
|
|
|
Supported private key types are RSA, ED25519, ECDSA, and ECDH.
|
|
|
|
It may be specified multiple times in a TLS URI.
|
|
*/
|
|
TlsUriParamKey string = "pki_key"
|
|
/*
|
|
TlsUriParamNoVerify, if `1`, `yes`, `y`, or `true` indicate
|
|
that the TLS connection should not require verification of
|
|
the remote end (e.g. hostname matches, trusted chain, etc.).
|
|
|
|
Any other value for this parameter will be parsed as "False"
|
|
(meaning the remote end's certificate SHOULD be verified).
|
|
|
|
Only the first defined instance is parsed.
|
|
*/
|
|
TlsUriParamNoVerify string = "no_verify"
|
|
/*
|
|
TlsUriParamSni indicates that the TLS connection should expect this hostname
|
|
instead of the hostname specified in the URI itself.
|
|
|
|
Only the first defined instance is parsed.
|
|
*/
|
|
TlsUriParamSni string = "sni"
|
|
/*
|
|
TlsUriParamCipher specifies one (or more) cipher(s)
|
|
to specify for the TLS connection cipher negotiation.
|
|
Note that TLS 1.3 has a fixed set of ciphers, and
|
|
this list may not be respected by the remote end.
|
|
|
|
The string may either be the name (as per
|
|
https://www.iana.org/assignments/tls-parameters/tls-parameters.xml)
|
|
or an int (normal, hex, etc. string representation).
|
|
|
|
It may be specified multiple times in a TLS URI.
|
|
*/
|
|
TlsUriParamCipher string = "cipher"
|
|
/*
|
|
TlsUriParamCurve specifies one (or more) curve(s)
|
|
to specify for the TLS connection cipher negotiation.
|
|
|
|
It may be specified multiple times in a TLS URI.
|
|
*/
|
|
TlsUriParamCurve string = "curve"
|
|
/*
|
|
TlsUriParamMinTls defines the minimum version of the
|
|
TLS protocol to use.
|
|
It is recommended to use "TLS_1.3".
|
|
|
|
Supported syntax formats include:
|
|
|
|
* TLS_1.3
|
|
* 1.3
|
|
* v1.3
|
|
* TLSv1.3
|
|
* 0x0304 (legacy_version, see RFC8446 § 4.1.2)
|
|
* 774 (0x0304 in int form)
|
|
* 0o1404 (0x0304 in octal form)
|
|
|
|
All evaluate to TLS 1.3 in this example.
|
|
|
|
Only the first defined instance is parsed.
|
|
*/
|
|
TlsUriParamMinTls string = "min_tls"
|
|
/*
|
|
TlsUriParamMaxTls defines the minimum version of the
|
|
TLS protocol to use.
|
|
|
|
See TlsUriParamMinTls for syntax of the value.
|
|
|
|
Only the first defined instance is parsed.
|
|
*/
|
|
TlsUriParamMaxTls string = "max_tls"
|
|
/*
|
|
TlsUriParamNet is used by TlsUri.ToConn and TlsUri.ToTlsConn to explicitly specify a network.
|
|
|
|
The default is "tcp".
|
|
|
|
See net.Dial()'s "network" parameter for valid network types.
|
|
|
|
Only the first defined instance is parsed.
|
|
*/
|
|
TlsUriParamNet string = "net"
|
|
)
|
|
|
|
var (
|
|
paramBoolValsTrue []string = []string{
|
|
"1", "yes", "y", "true",
|
|
}
|
|
paramBoolValsFalse []string = []string{
|
|
"0", "no", "n", "false",
|
|
}
|
|
validate *validator.Validate = validator.New(validator.WithRequiredStructEnabled())
|
|
)
|